Uncomplicate JavaScript
Deno is the open-source JavaScript runtime for the modern web. Built on web standards with zero-config TypeScript, unmatched security, and a complete built-in toolchain, Deno is the easiest, most productive way to JavaScript.
- Rating90k+Stars on GitHub
- Community250k+Active Deno users
- Ecosystem2M+Community modules
Native TypeScript support
interface Person {
name: string;
age: number;
}
function greet(person: Person): string {
return "Hello, " + person.name + "!";
}
const alice: Person = {
name: "Alice",
age: 36
};
console.log(greet(alice));
Modern language features, built-in
Built on web standards
Consistent code from browser to backend
Batteries included
The essential tools you need to build, test, and deploy your applications are all included out of the box.
Code linter
$ deno lint --watch
Deno ships with a built-in code linter to help you avoid bugs and code rot.
Learn moreStandalone executables
Deno.serve(req => new Response("Hello!"));
$ deno compile --allow-net server.ts
$ ./server
Listening on http://localhost:8000/
Instantly create standalone executables from your Deno program. It even supports cross-compiling for other platforms!
Learn moreTest runner
$ deno test main_test.ts
Deno.test("1 + 2 = 3", () => {
const x = 1 + 2;
console.assert(x == 3);
});
Deno provides a test runner and assertion libraries as a part of the runtime and standard library.
Learn moreCode formatter
$ deno fmt --line-width=120
Deno's built-in code formatter (based on dprint) beautifies JavaScript, TypeScript, JSON, and Markdown.
Learn moreSecure by default
Prevent supply chain attacks
Stop worrying about npm modules introducing unexpected vulnerabilities. Deno restricts access to the file system, network, and system environment by default, so code can access only what you allow.
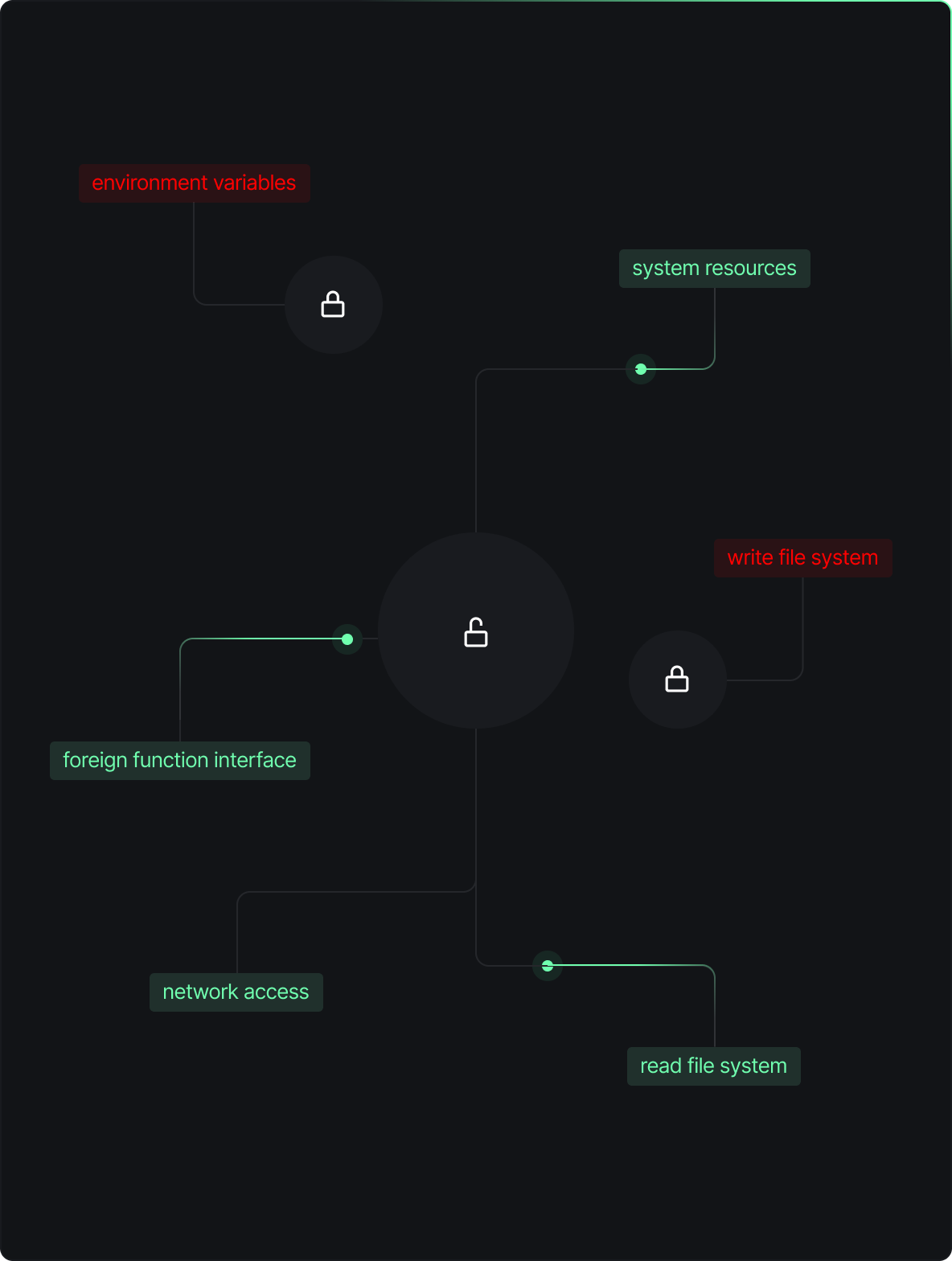
Backwards compatible with Node.js
import express from "npm:express@4";
const app = express();
app.get("/", function (_req, res) {
res.send("hello");
});
app.listen(3000, () => {
console.log("Express listening on :3000");
});
$ deno run --allow-net --allow-read --allow-env server.js
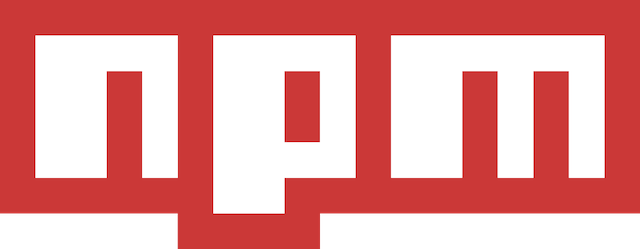
Compatible with millions of npm modules
npm:
specifiers.High-performance networking
- HTTPS (encryption)
- WebSocket
- HTTP2
- Automatic response body compression
Deno.serve(req => new Response("Hello world"));
Bigger is better
Throughput, requests per sec
Built for the cloud
Deno runs on
Go further with Deno cloud products
Deno Deploy
For developers looking for the simplest way to host web apps and APIs
- Fully managed serverless solution
- Globally distributed
- Built-in key/value database, queues, cron, and more
- Integrated directly with GitHub
Deno Subhosting
For SaaS companies looking to run user code securely
- Secure sandboxed functions
- Automatic scaling and provisioning
- Globally distributed
- Manage via a simple API
Seamless persistence with Deno KV
The Deno runtime ships with Deno KV, a key/value database designed for globally distributed applications.
Go from development to production on Deno Deploy with no API keys or infrastructure to configure.
The Freshest web framework
Build fast sites fast
export default function HomePage() {
return (
<div>
<h1>HTML fresh from the server!</h1>
<p>
Delivered at
{new Date().toLocaleTimeString()}
</p>
</div>
);
}
import { useSignal } from "@preact/signals";
export default function Counter() {
const count = useSignal<number>(0);
return (
<button onClick={() => count.value += 1}>
The count is {count.value}
</button>
);
}
Ship less JavaScript
Our vibrant community
“I know this was gonna happen! Deno is truly building the fastest, most secure and personalizable JS runtime!”
“Deno's security model is PERFECT for this type of script. Running a script from a rando off the internet? It asks for read access to only the CWD and then asks for access to the file it wants to write to. 👏”
“I really think Deno is the easiest and most capable JS runtime. URL imports are slept on.”
“npm packages in Deno 👀 That’s an exciting development for those of us building at the edge.”
“This Deno thing is fast, no doubt about it. #denoland”
“Deno: I have to use the browser APIs cause they are everywhere, and everywhere is my target runtime (the web). The runtime that tries to mirror browser APIs server side makes my life easiest.”
“Deno is fantastic. I am using it to level up a bit in terms of JavaScript and TypeScript and it is the easiest way to get going. Their tooling is like 100x simpler than all the usual Node stacks.”